JAVA Knowledge Base
Wednesday, December 18, 2024
Understanding Essential DNS Record Types for Web Administrators
Production release deployment strategies
Production release deployment strategies
The five deployment strategies for software delivery:
1. Blue/Green Deployment:
• Two environments: Blue (current, active) and Green (new version).
• Traffic is switched to the Green environment after deployment and testing.
• The Blue environment acts as a fallback if issues occur.
2. Canary Deployment:
• New version is rolled out gradually.
• A small percentage of traffic (e.g., 25%) is initially sent to the new version.
• Once stable, more traffic is routed to the new version (e.g., 75%).
3. A/B Testing:
• Two versions (e.g., V1 and V2) run simultaneously for different user groups.
• Helps determine which version performs better based on user feedback or metrics.
4. Feature Flag:
• Feature toggles control new features.
• New features can be enabled for specific users (e.g., 20%) without deploying new code.
5. Rolling Deployment:
• New version is rolled out in stages (e.g., Stage 0 to Stage 3).
• Gradually replaces instances of the old version without downtime.
Tuesday, December 17, 2024
The Ultimate Roadmap to Learning Python Programming in 2024
The Ultimate Roadmap to Learning Python Programming in 2024
Monday, December 16, 2024
Top API Security Best Practices to Protect Your Application
Top API Security Best Practices to Protect Your Application
REST API Application Methods
REST API Application Methods
Tuesday, December 03, 2024
Microservices Design Patterns
Microservices Design Pattern
Monday, December 02, 2024
Mastering Event-Driven Architectural Patterns: A Comprehensive Guide
Mastering Event-Driven Architectural Patterns: A Comprehensive Guide
Event-driven architecture (EDA) is an essential paradigm for building scalable, resilient, and decoupled systems. By enabling asynchronous communication and handling real-time events, EDA empowers systems to respond to user actions, system events, and data changes effectively. Below is an overview of common event-driven architectural patterns and how they function.
1. Competing Consumer Pattern
Overview:
This pattern involves multiple consumers listening to a message queue, competing to process messages. This allows for parallel processing and better resource utilization.
Use Case:
Useful when you have tasks that can be processed in parallel, such as handling customer orders or processing transactions.
Key Benefits:
- Load balancing among consumers.
- Improved throughput by parallelizing tasks.
- Scalability through horizontal scaling of consumers.
2. Consume and Project Pattern
Overview:
This pattern separates the concerns of reading and writing by creating materialized views that are projections of the event data. The system listens to events and updates a view model accordingly.
Use Case:
Generating read-optimized data models from event streams, such as maintaining customer order views.
Key Benefits:
- Optimized reads for fast query performance.
- Decouples read operations from the primary service.
- Easier to create customized views based on business needs.
3. Event Sourcing
Overview:
Instead of storing the current state, this pattern persists all changes (events) that lead to the current state. The state can be reconstructed by replaying these events.
Use Case:
Building audit logs or systems where tracking every state change is critical, such as financial systems.
Key Benefits:
- Complete audit trail of all changes.
- Easy to debug or replay events.
- Enables time-travel scenarios (state reconstruction).
4. Async Task Execution Pattern
Overview:
Tasks are dispatched to workers asynchronously, often categorized by priority. A queue manages these tasks, and workers consume them accordingly.
Use Case:
Handling background jobs like image processing, email notifications, or report generation.
Key Benefits:
- Offloads long-running tasks from the main thread.
- Ensures task prioritization through separate queues.
- Increases system responsiveness by delegating tasks.
5. Transactional Outbox Pattern
Overview:
This pattern ensures that updates to the database and the corresponding event publication happen atomically. The system writes both the state change and the event to an outbox table in the same transaction.
Use Case:
Guaranteeing consistency between the database and message queue, particularly in distributed systems.
Key Benefits:
- Avoids message loss due to transaction failure.
- Ensures data consistency across services.
- Simplifies rollback mechanisms.
6. Event Aggregation Pattern
Overview:
Fine-grained events from various sources are aggregated into a single, coarser-grained event. An event aggregator service performs this task.
Use Case:
Aggregating customer-related events (e.g., contact creation, account updates) into a unified customer creation event.
Key Benefits:
- Reduces the number of events downstream services need to process.
- Simplifies event processing logic.
- Enables consolidation of related data into meaningful events.
7. Saga Pattern
Overview:
The saga pattern manages distributed transactions by coordinating a series of local transactions. Each step in the saga emits an event that triggers the next step.
Use Case:
Orchestrating complex business workflows, such as order processing across multiple services.
Key Benefits:
- Ensures eventual consistency in distributed systems.
- Handles partial failures gracefully with compensating actions.
- Simplifies complex workflows by breaking them into smaller steps.
Conclusion
Event-driven architectural patterns are critical for designing robust, scalable, and decoupled systems. Each pattern addresses specific challenges, from improving scalability with competing consumers to ensuring data consistency with the transactional outbox. By mastering these patterns, you can build systems that are both responsive and resilient, enabling your applications to handle real-time events efficiently.
Understanding Essential DNS Record Types for Web Administrators
Understanding Essential DNS Record Types for Web Administrators Introduction The Domain Name System (DNS) acts as the backbone of the inte...
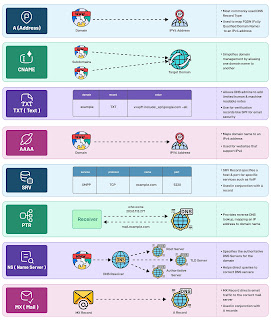
-
Kafka Use cases Use cases of Apache Kafka , a distributed event-streaming platform. Let’s walk through each section in detail: 1. Stre...
-
Hi Those who want to learn hadoop, Initially start with the below hadoop tutorial links https://hadoop.apache.org/docs/r1.2.1/mapred_...
-
Seamless Integration: A Complete Guide to Using the ChatGPT API in Your Applications Here’s how you can make a cURL request for generating...